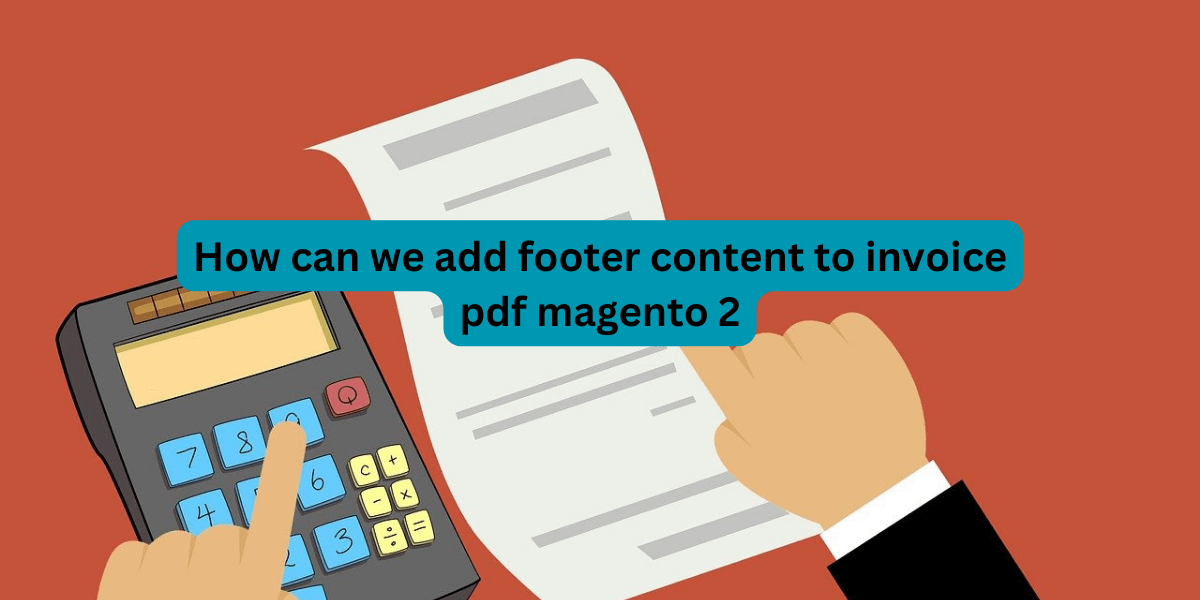
In the world of e-commerce, every interaction with a customer is an opportunity to reinforce your brand identity and provide valuable information. One often overlooked area for customization is the invoice. While the default Magento 2 invoice layout is functional, adding a custom footer can elevate the user experience and provide additional information or branding.
Magento 2, being a highly customizable platform, offers several methods to add custom footer content to your invoices. In this guide, we'll explore some of the most effective ways to achieve this.
First, let's create a custom module to handle the customization of the invoice PDF.
app/code/Mageefy/CustomizeInvoice/etc/di.xml
<?xml version="1.0"?>
<!--
/**
* @category Mageefy
* @package Mageefy_CustomizeInvoice
* @author Mageefy Extension Team
* @copyright Copyright (c) Mageefy ( https://www.mageefy.com )
* @license https://www.mageefy.com/license-agreement
*/
-->
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<preference for="Magento\Sales\Model\Order\Pdf\Invoice" type="Mageefy\CustomizeInvoice\Rewrite\Magento\Sales\Model\Order\Pdf\Invoice"/>
</config>
app/code/Mageefy/CustomizeInvoice/Rewrite/Magento/Sales/Model/Order/Pdf/Invoice.php
<?php
/**
* @category Mageefy
* @package Mageefy_CustomizeInvoice
* @author Mageefy Extension Team
* @copyright Copyright (c) Mageefy ( https://www.mageefy.com )
* @license https://www.mageefy.com/license-agreement
*/
namespace Mageefy\CustomizeInvoice\Rewrite\Magento\Sales\Model\Order\Pdf;
use Magento\Framework\View\LayoutInterface;
use Magento\Sales\Model\Order\Pdf\Config;
use Magento\Sales\Model\Order\Pdf\Invoice as BaseInvoice;
class Invoice extends BaseInvoice
{
protected $_layout;
/**
* Invoice constructor.
* @param \Magento\Payment\Helper\Data $paymentData
* @param \Magento\Framework\Stdlib\StringUtils $string
* @param \Magento\Framework\App\Config\ScopeConfigInterface $scopeConfig
* @param \Magento\Framework\Filesystem $filesystem
* @param Config $pdfConfig
* @param \Magento\Sales\Model\Order\Pdf\Total\Factory $pdfTotalFactory
* @param \Magento\Sales\Model\Order\Pdf\ItemsFactory $pdfItemsFactory
* @param \Magento\Framework\Stdlib\DateTime\TimezoneInterface $localeDate
* @param \Magento\Framework\Translate\Inline\StateInterface $inlineTranslation
* @param \Magento\Sales\Model\Order\Address\Renderer $addressRenderer
* @param \Magento\Store\Model\StoreManagerInterface $storeManager
* @param \Magento\Framework\Locale\ResolverInterface $localeResolver
* @param LayoutInterface $layout
* @param array $data
*/
public function __construct(
\Magento\Payment\Helper\Data $paymentData,
\Magento\Framework\Stdlib\StringUtils $string,
\Magento\Framework\App\Config\ScopeConfigInterface $scopeConfig,
\Magento\Framework\Filesystem $filesystem,
Config $pdfConfig,
\Magento\Sales\Model\Order\Pdf\Total\Factory $pdfTotalFactory,
\Magento\Sales\Model\Order\Pdf\ItemsFactory $pdfItemsFactory,
\Magento\Framework\Stdlib\DateTime\TimezoneInterface $localeDate,
\Magento\Framework\Translate\Inline\StateInterface $inlineTranslation,
\Magento\Sales\Model\Order\Address\Renderer $addressRenderer,
\Magento\Store\Model\StoreManagerInterface $storeManager,
\Magento\Framework\Locale\ResolverInterface $localeResolver,
LayoutInterface $layout,
array $data = []
) {
$this->_layout = $layout;
parent::__construct(
$paymentData,
$string,
$scopeConfig,
$filesystem,
$pdfConfig,
$pdfTotalFactory,
$pdfItemsFactory,
$localeDate,
$inlineTranslation,
$addressRenderer,
$storeManager,
$localeResolver,
$data
);
}
/**
* @param array $invoices
* @return \Zend_Pdf
* @throws \Zend_Pdf_Exception
*/
public function getPdf($invoices = [])
{
$this->_beforeGetPdf();
$this->_initRenderer("invoice");
$pdf = new \Zend_Pdf();
$this->_setPdf($pdf);
$style = new \Zend_Pdf_Style();
$this->_setFontBold($style, 10);
foreach ($invoices as $invoice) {
if ($invoice->getStoreId()) {
$this->_localeResolver->emulate($invoice->getStoreId());
$this->_storeManager->setCurrentStore($invoice->getStoreId());
}
$page = $this->newPage();
$order = $invoice->getOrder();
/* Add image */
$this->insertLogo($page, $invoice->getStore());
/* Add address */
$this->insertAddress($page, $invoice->getStore());
/* Add head */
$this->insertOrder(
$page,
$order,
$this->_scopeConfig->isSetFlag(
self::XML_PATH_SALES_PDF_INVOICE_PUT_ORDER_ID,
\Magento\Store\Model\ScopeInterface::SCOPE_STORE,
$order->getStoreId()
)
);
/* Add document text and number */
$this->insertDocumentNumber(
$page,
__("Invoice # ") . $invoice->getIncrementId()
);
/* Add table */
$this->_drawHeader($page);
/* Add body */
foreach ($invoice->getAllItems() as $item) {
if ($item->getOrderItem()->getParentItem()) {
continue;
}
/* Draw item */
$this->_drawItem($item, $page, $order);
$page = end($pdf->pages);
}
/* Add totals */
$this->insertTotals($page, $invoice);
if ($invoice->getStoreId()) {
$this->_localeResolver->revert();
}
/* Add footer content */
$this->drawFooter($page);
}
$this->_afterGetPdf();
return $pdf;
}
/**
* @param \Zend_Pdf_Page $page
* @param String $value
*/
public function drawFooter(\Zend_Pdf_Page $page)
{
try {
$font = $this->_setFontRegular($page, 10);
$this->y -= 10;
$value = $this->getFooterContent();
$line = 52;
if ($value !== "") {
$value = preg_replace("/<br[^>]*>/i", "\n", $value);
$page->setFillColor(new \Zend_Pdf_Color_RGB(0, 0, 0));
$page->setLineColor(new \Zend_Pdf_Color_GrayScale(0.5));
foreach (explode("\n", $value) as $textLine) {
$feed = $this->getAlignCenter(
$textLine,
30,
520,
$font,
10
);
$page->drawText(
strip_tags($textLine),
$feed,
$line,
"UTF-8"
);
$line -= 16;
}
$page->setFillColor(new \Zend_Pdf_Color_GrayScale(0));
}
$this->y -= 20;
} catch (\Exception $e) {
$this->_logger->critical($e);
}
}
/**
* @return mixed
*/
public function getFooterContent()
{
if (
$footerCms = $this->_layout
->createBlock("Magento\Cms\Block\Block")
->setBlockId("pdf_invoice_text")
) {
return $footerCms->toHtml();
}
}
}
By following these steps, you can programmatically add custom footer content to your Magento 2 invoice PDF. This allows you to tailor the invoice PDF to better suit your branding and communication needs, providing a more professional and personalized experience for your customers.