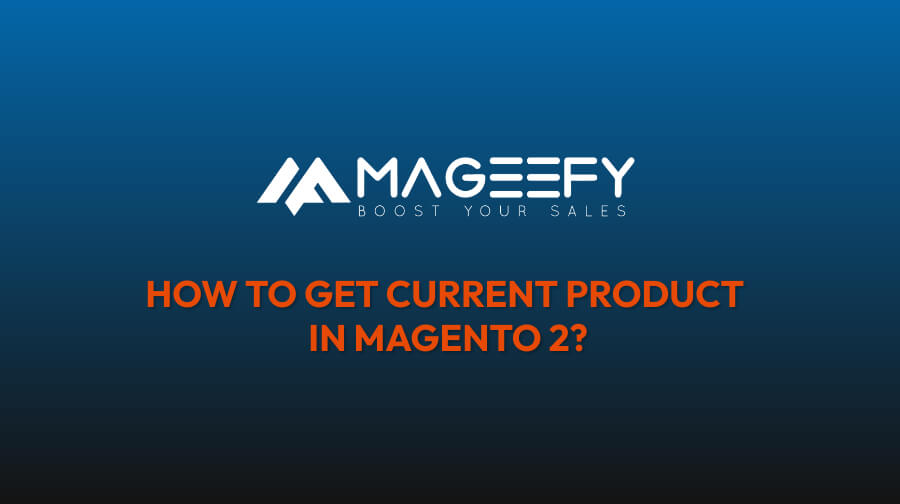
If you are working on any Magento 2 project, then many times you need to get current product object. Magento 2 has several different ways to get these data. Here we are going explain more popular ways. Magento allows you to create and configure 6 different product types in the admin panel. To get current product details you need to use Registry. The current product in Magento 2 is stored to Magento registry, with the key current_product. The current product includes the following information: name, sku, final price, url and associated category ids. Use below code snippet to get the current product.
Use the below code in your block file.
<?php
namespace Mageefy\Custom\Block:
class BlockClass extends \Magento\Framework\View\Element\Template
{
protected $registry;
public function __construct(
\Magento\Backend\Block\Template\Context $context,
\Magento\Framework\Registry $registry,
array $data = []
)
{
$this->registry = $registry;
parent::__construct($context, $data);
}
public function _prepareLayout()
{
return parent::_prepareLayout();
}
public function getCurrentProduct()
{
return $this->_registry->registry('current_product');
}
}
?>
Call function in your .phtml file:
<?php
$currentProduct = $block->getCurrentProduct();
echo $currentProduct->getId() . '<br />';
echo $currentProduct->getName() . '<br />';
echo $currentProduct->getSku() . '<br />';
echo $currentProduct->getFinalPrice() . '<br />';
echo $currentProduct->getProductUrl() . '<br />';
print_r ($currentProduct->getCategoryIds()) . '<br />';
?>
If you have any queries about the article or any questions, use the comment section below.