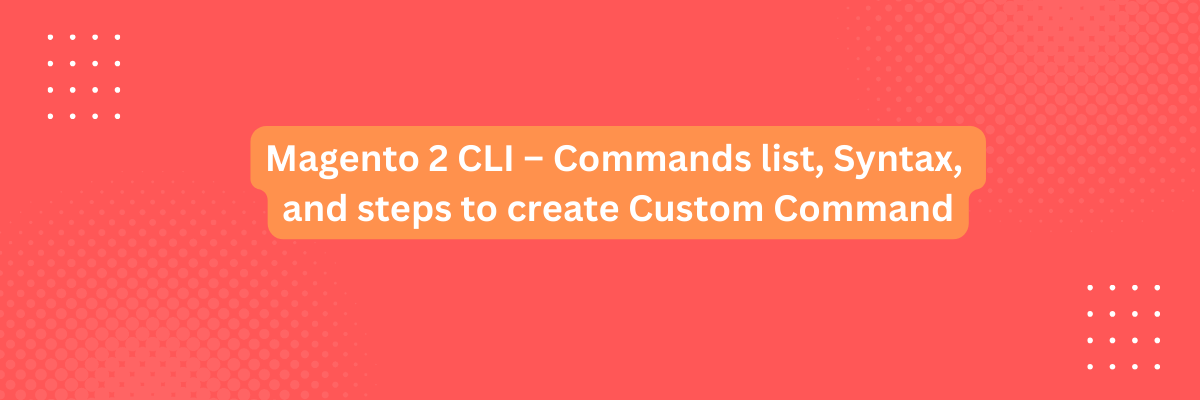
Magento 2 provides a Command Line Interface (CLI) that allows you to perform various tasks, manage your store, and interact with your Magento installation. You can also create custom CLI commands in Magento 2. Below, I'll provide you with a list of common Magento 2 CLI commands, the syntax for running them, and the steps to create a custom CLI command.
Common Magento 2 CLI Commands:
Here is a list of some common Magento 2 CLI commands:
-
Cache Management:
- Command:
bin/magento cache:clean
- Description: Clean the cache.
- Command:
-
Module Management:
- Command:
bin/magento module:status
- Description: Check the status of modules.
- Command:
-
Setup Commands:
- Command:
bin/magento setup:upgrade
- Description: Upgrade the Magento application.
- Command:
-
Index Management:
- Command:
bin/magento indexer:reindex
- Description: Reindex all Magento indexers.
- Command:
-
Database Operations:
- Command:
bin/magento setup:db-schema:upgrade
- Description: Upgrade the database schema.
- Command:
-
Store Configuration:
- Command:
bin/magento config:set <path> <value>
- Description: Set a configuration value.
- Command:
-
User Management:
- Command:
bin/magento admin:user:create
- Description: Create a new admin user.
- Command:
-
Customer Management:
- Command:
bin/magento customer:create
- Description: Create a new customer.
- Command:
Magento 2 CLI Command Syntax:
The basic syntax for running Magento 2 CLI commands is as follows:
bin/magento <command> [options] [arguments]
<command>
: The name of the command you want to execute.[options]
: Optional flags or options that modify the behavior of the command.[arguments]
: Optional arguments that the command may require.
Steps to Create a Custom Magento 2 CLI Command:
To create a custom CLI command in Magento 2, follow these steps:
-
Create a new module:
- Create a new module or use an existing one to contain your custom command.
-
Create a command class:
- Create a PHP class that extends
\Symfony\Component\Console\Command\Command
. This class will define your custom command's behavior.
- Create a PHP class that extends
-
Implement the required methods:
- In your custom command class, implement the
configure()
andexecute()
methods. Theconfigure()
method is used to define the command's name, description, and any required options or arguments. Theexecute()
method contains the logic for your command.
- In your custom command class, implement the
-
Register the command:
- Register your custom command class in your module's
di.xml
file to make it available as a CLI command.
- Register your custom command class in your module's
-
Run the command:
- After registering your command, you can run it using the
bin/magento
command-line tool.
- After registering your command, you can run it using the
Here's a simplified example of what your custom command class might look like:
<?php
namespace YourNamespace\YourModule\Console\Command;
use Symfony\Component\Console\Command\Command;
use Symfony\Component\Console\Input\InputInterface;
use Symfony\Component\Console\Output\OutputInterface;
class YourCustomCommand extends Command
{
protected function configure()
{
$this->setName('yourmodule:customcommand')
->setDescription('Description of your custom command');
}
protected function execute(InputInterface $input, OutputInterface $output)
{
// Your custom command logic goes here
$output->writeln('Your custom command executed.');
}
}
Remember to replace YourNamespace
, YourModule
, and customize the command name, description, and logic as needed.
By following these steps, you can create and execute custom CLI commands in Magento 2.