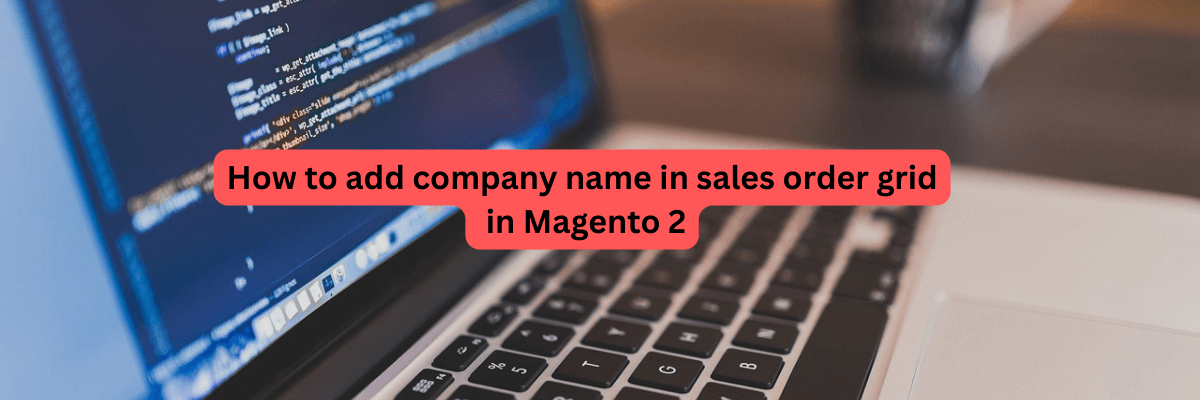
Magento 2 is a powerful e-commerce platform that provides extensive customization options for online store owners. One common requirement for businesses is to display the company name in the sales order grid for better organization and management. In this tutorial, we will guide you through the process of adding the company name column with filter to the sales order grid in Magento 2.
Step 1: Create a Custom Module
First, let's create a custom module to handle our modifications. In your Magento 2 root directory, navigate to app/code and create a new directory for your module. For example, if your company is "Mageefy" the directory structure would be app/code/Mageefy/OrderGridCompany
Inside the module directory, create the following files:
app/code/Mageefy/OrderGridCompany/registration.php
app/code/Mageefy/OrderGridCompany/etc/module.xml
Add the necessary module registration information in these files.
Step 2: Create a UI Component to Display the Company Name
Now, we need to create a UI component to display the company name column in the sales order grid. Create a new file named sales_order_grid.xml in the view/adminhtml/ui_component directory of your module:
<!-- app/code/Mageefy/OrderGridCompany/view/adminhtml/ui_component/sales_order_grid.xml -->
<?xml version="1.0" encoding="UTF-8"?>
<listing xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Ui:etc/ui_configuration.xsd">
<columns name="sales_order_columns">
<column name="company">
<argument name="data" xsi:type="array">
<item name="config" xsi:type="array">
<item name="component" xsi:type="string">Magento_Ui/js/grid/columns/column</item>
<item name="label" xsi:type="string" translate="true">Company</item>
<item name="sortOrder" xsi:type="number">60</item>
<item name="align" xsi:type="string">left</item>
<item name="dataType" xsi:type="string">text</item>
<item name="visible" xsi:type="boolean">true</item>
<item name="filter" xsi:type="string">text</item>
</item>
</argument>
</column>
</columns>
</listing>
This XML file configures the new column, specifying its name, class, and label.
Step 3: Create di.xml file inside etc directory of your module and add below code
<type name="Magento\Framework\View\Element\UiComponent\DataProvider\CollectionFactory">
<plugin name="mageefy_sales_order_additional_columns_company"
type="Mageefy\OrderGridCompany\Plugin\OrdersGrid"
sortOrder="20"
disabled="false"/>
</type>
Step 3: Create a plugin that feeds the column with the value from the sales_order_address table
Create a new file named OrdersGrid.php in the Plugin directory of your module and paste below code
<?php
/**
* @category Mageefy
* @package Mageefy_OrderGridCompany
* @author Mageefy Extension Team
* @copyright Copyright (c) Mageefy ( https://www.mageefy.com )
* @license https://www.mageefy.com/license-agreement
*/
namespace Mageefy\OrderGridCompany\Plugin;
use Magento\Sales\Model\ResourceModel\Order\Grid\Collection as OrderGridCollection;
/**
* Class OrdersGrid
*/
class OrdersGrid
{
/**
* @var LoggerInterface
*/
private $logger;
/**
* @param Logger $logger
*/
public function __construct(
\Psr\Log\LoggerInterface $customLogger,
array $data = []
) {
$this->logger = $customLogger;
}
public function afterGetReport($subject, $collection, $requestName)
{
if ($requestName !== 'sales_order_grid_data_source') {
return $collection;
}
if ($collection->getMainTable() === $collection->getResource()->getTable('sales_order_grid')) {
try {
$orderAddressTable = $collection->getResource()->getTable('sales_order_address');
$collection->getSelect()->joinLeft(
['oat' => $orderAddressTable],
'oat.parent_id = main_table.entity_id AND oat.address_type = "billing"',
['company']
);
} catch (Zend_Db_Select_Exception $selectException) {
$this->logger->log(100, $selectException);
}
}
return $collection;
}
}
To complete the process, execute the setup:di:compile command. Ensure that you refresh the configuration cache after making modifications to XML files. We hope this is useful for you. Feel free to share any feedback in the comments section.