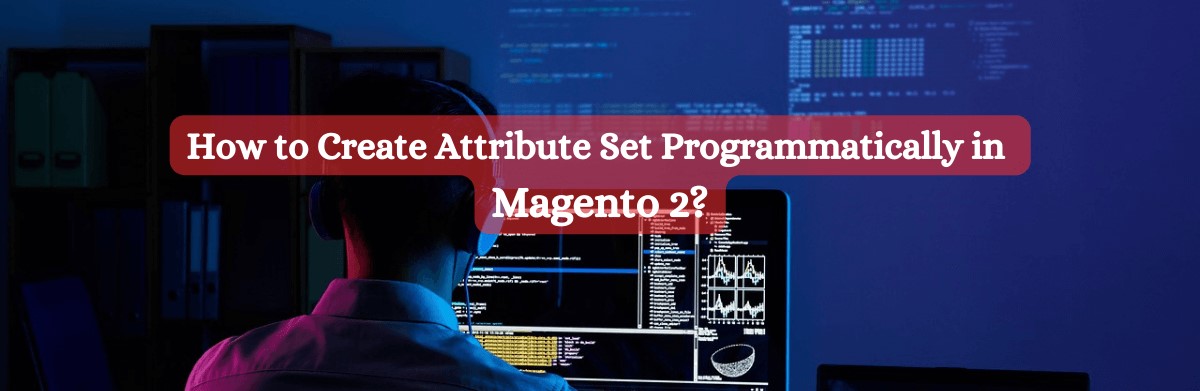
Magento 2 is a powerful e-commerce platform that offers extensive customization options to meet the unique requirements of online businesses. One of the key features of Magento 2 is its flexibility in managing product attributes and attribute sets. In this blog post, we will explore the process of creating attribute sets programmatically in Magento 2, allowing you to tailor your product attributes to match your specific business needs.
Why Attribute Sets Matter:
Attribute sets in Magento 2 play a crucial role in organizing and categorizing your products. They define the collection of attributes that are applicable to a particular type of product. By creating custom attribute sets, you can ensure that the product information is structured in a way that aligns with your business model, making it easier for customers to navigate and find the products they are looking for.
Use the following code to create an attribute set programmatically.
Create the InstallData.php file in the app/code/Mageefy/Custom/Setup/InstallData.php.
Here, Vendor Name is Mageefy and Module Name is Custom.
<?php
namespace Mageefy\Custom\Setup;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
use Magento\Catalog\Setup\CategorySetupFactory;
use Magento\Eav\Model\Entity\Attribute\SetFactory as AttributeSetFactory;
class InstallData implements InstallDataInterface
{
private $attributeSetFactory;
private $attributeSet;
private $categorySetupFactory;
public function __construct(AttributeSetFactory $attributeSetFactory, CategorySetupFactory $categorySetupFactory )
{
$this->attributeSetFactory = $attributeSetFactory;
$this->categorySetupFactory = $categorySetupFactory;
}
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$setup->startSetup();
$categorySetup = $this->categorySetupFactory->create(['setup' => $setup]);
$attributeSet = $this->attributeSetFactory->create();
$entityTypeId = $categorySetup->getEntityTypeId(\Magento\Catalog\Model\Product::ENTITY);
$attributeSetId = $categorySetup->getDefaultAttributeSetId($entityTypeId);
$data = [
'attribute_set_name' => 'MyCustomAttribute',
'entity_type_id' => $entityTypeId,
'sort_order' => 400,
];
$attributeSet->setData($data);
$attributeSet->validate();
$attributeSet->save();
$attributeSet->initFromSkeleton($attributeSetId);
$attributeSet->save();
$setup->endSetup();
}
}
Finally, test your code by executing it and verifying that the attribute set is created successfully. Check the Magento 2 admin panel to ensure that the attribute set appears with the assigned attributes.