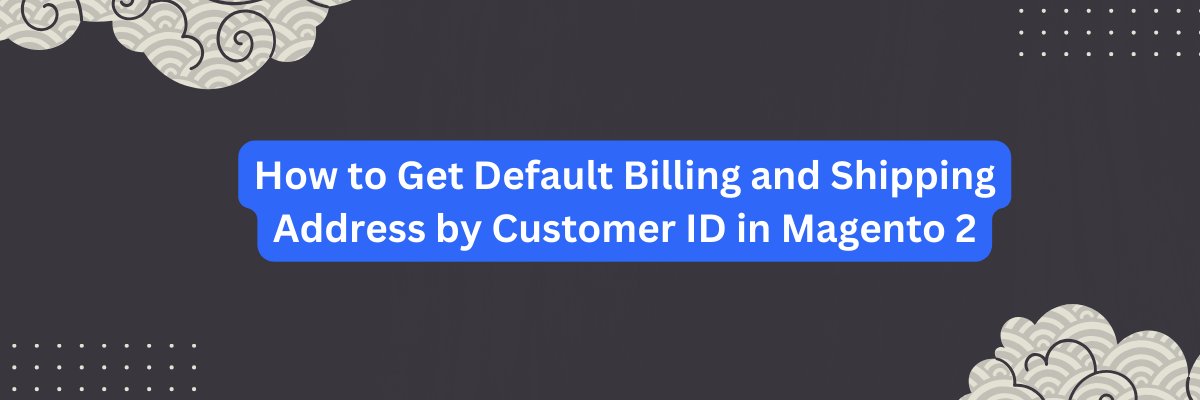
In Magento 2, you can retrieve the default billing and shipping addresses for a customer by their customer ID. You can do this by using Magento's built-in dependency injection and methods. Here's a step-by-step guide on how to accomplish this:
-
Create a custom PHP class or function where you intend to retrieve the default billing and shipping addresses. You can place this code in a custom module, a controller, a block class, or any other suitable location within your Magento 2 installation.
-
Inject the necessary dependencies into your class or function. You'll need the following dependencies:
\Magento\Customer\Api\CustomerRepositoryInterface
,\Magento\Customer\Model\AddressFactory
, and\Magento\Customer\Api\AddressRepositoryInterface
. You can do this through constructor injection.
Here's an example of how you can inject these dependencies:
use Magento\Customer\Api\CustomerRepositoryInterface;
use Magento\Customer\Model\AddressFactory;
use Magento\Customer\Api\AddressRepositoryInterface;
class YourCustomClass
{
protected $customerRepository;
protected $addressFactory;
protected $addressRepository;
public function __construct(
CustomerRepositoryInterface $customerRepository,
AddressFactory $addressFactory,
AddressRepositoryInterface $addressRepository
) {
$this->customerRepository = $customerRepository;
$this->addressFactory = $addressFactory;
$this->addressRepository = $addressRepository;
}
// Your methods to retrieve default billing and shipping addresses go here
}
1. Create methods to retrieve the default billing and shipping addresses for a customer by their ID. You can use the customer ID to load the customer object and then retrieve their default addresses.
public function getDefaultBillingAddressByCustomerId($customerId)
{
$customer = $this->customerRepository->getById($customerId);
$defaultBillingAddressId = $customer->getDefaultBilling();
if ($defaultBillingAddressId) {
return $this->addressRepository->getById($defaultBillingAddressId);
}
return null; // No default billing address found
}
public function getDefaultShippingAddressByCustomerId($customerId)
{
$customer = $this->customerRepository->getById($customerId);
$defaultShippingAddressId = $customer->getDefaultShipping();
if ($defaultShippingAddressId) {
return $this->addressRepository->getById($defaultShippingAddressId);
}
return null; // No default shipping address found
}
Now, you can use these methods to retrieve the default billing and shipping addresses for a customer by their ID. For example:
$customerId = 1; // Replace with the actual customer ID
$billingAddress = $this->getDefaultBillingAddressByCustomerId($customerId);
$shippingAddress = $this->getDefaultShippingAddressByCustomerId($customerId);
if ($billingAddress) {
// Do something with the default billing address
}
if ($shippingAddress) {
// Do something with the default shipping address
}
Make sure to replace YourCustomClass
with the actual name of your custom class or function, and replace $customerId
with the customer ID for which you want to retrieve the addresses.
This code will allow you to retrieve the default billing and shipping addresses for a customer in Magento 2 using their customer ID.